Setup GitHub action for auto-deploying to an EC2
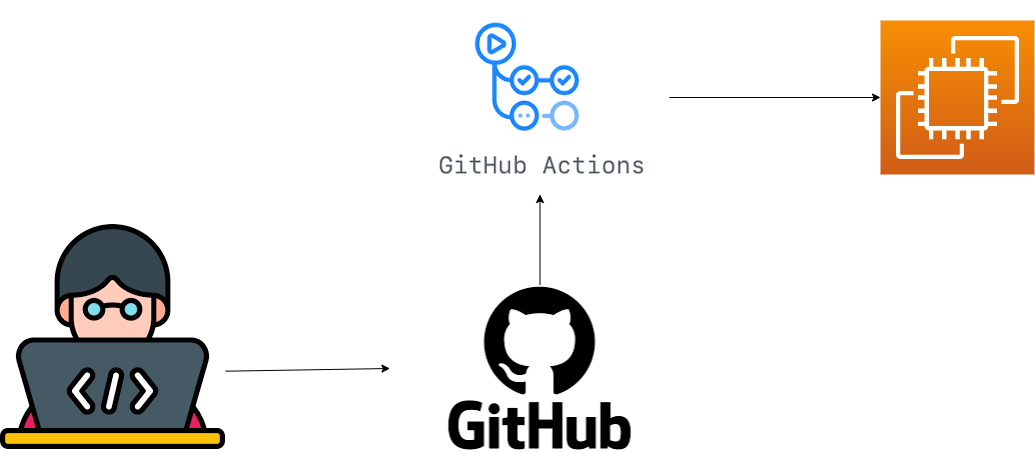
1. Generate ssh key on your EC2 then register to GitHub
1.1 Generate ssh key
# ssh to your ec2
$ ssh ec2
# move to .ssh folder
$ cd ~/.ssh
# generate key
$ ssh-keygen -t rsa
Enter file in which to save the key (): // github_key
Enter passphrase (empty for no passphrase): // Enter
Enter same passphrase again: // Enter
Create .ssh/config
file
Host github
HostName github.com
User git
IdentityFile ~/.ssh/github_key
1.2 Register ssh key
- Show the content of
github_key.pub
cat ~/.ssh/github_key.pub
- Copy the content of
github_key.pub
then add to authorized_keys
vim ~/.ssh/authorized_keys
- Copy the content of
github_key.pub
then register to githug
Go to https://github.com/settings/keys
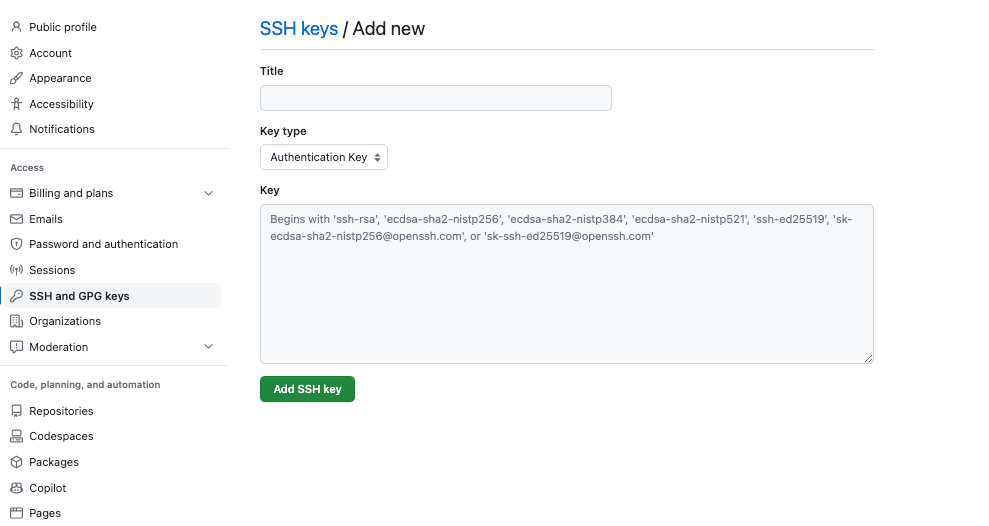
- Register ssh-agent
$ eval `ssh-agent`
$ ssh-add ~/.ssh/github_key
2. Create accessKey/secretKey
Create accessKey/secretKey with bellow policy
{
"Version": "2012-10-17",
"Statement": [
{
"Sid": "VisualEditor0",
"Effect": "Allow",
"Action": [
"ec2:RevokeSecurityGroupIngress",
"ec2:AuthorizeSecurityGroupIngress"
],
"Resource": "*"
}
]
}
3. Register GitHub repository secret:
AWS_ACCESS_KEY
<-— accessKey created in step 2
AWS_SECRET_ACCESS_KEY
<-— secretKey created in step 2
GIT_PRIVATE_KEY
<-— ~/.ssh/github_key created in step 1
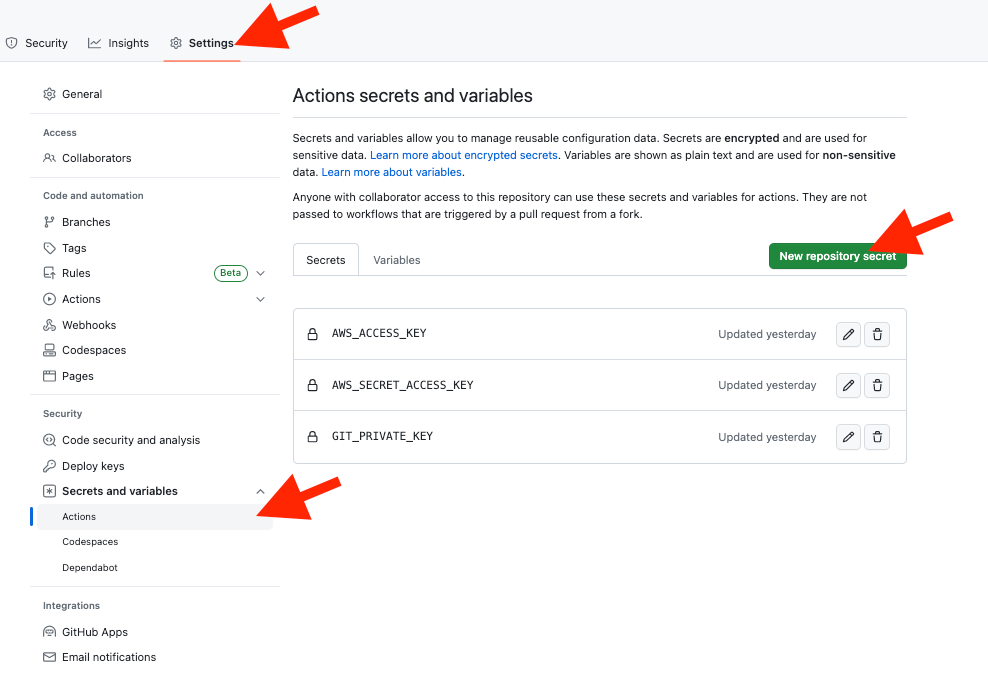
4. Create GitHub action workflows file
.github/workflows/test.yml
name: EC2 auto deploy TEST
on:
push:
branches: [ test ]
workflow_dispatch:
env:
EC2_USER_NAME: ec2-user
EC2_HOST_NAME: xx.xx.xx.xx
GIT_BRANCH: test
EC2_SECURITY_GROUP_ID: sg-xxx
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Public IP Install
id: ip
uses: haythem/public-ip@v1.2
- name: Checkout
uses: actions/checkout@v2
- name: AWS CLI install
run: |
curl "https://awscli.amazonaws.com/awscli-exe-linux-x86_64.zip" -o "awscliv2.zip"
unzip awscliv2.zip
sudo ./aws/install --update
aws --version
- name: AWS set Credentials
uses: aws-actions/configure-aws-credentials@v1
with:
aws-access-key-id: ${{ secrets.AWS_ACCESS_KEY }}
aws-secret-access-key: ${{ secrets.AWS_SECRET_ACCESS_KEY }}
aws-region: ap-northeast-1
- name: Add IP to security group
run: |
aws ec2 authorize-security-group-ingress --group-id ${{ env.EC2_SECURITY_GROUP_ID }} --protocol tcp --port 22 --cidr ${{ steps.ip.outputs.ipv4 }}/32
- name: Deploy
if: always()
run: |
# SSH then git pull
echo "${{ secrets.GIT_PRIVATE_KEY }}" > private_key
chmod 600 private_key
ssh -oStrictHostKeyChecking=no ${{ env.EC2_USER_NAME }}@${{ env.EC2_HOST_NAME }} -i private_key "cd /var/www/html/ && sudo git checkout ${{ env.GIT_BRANCH }} && git pull origin ${{ env.GIT_BRANCH }} && echo 'some command you want to run' "
# close ssh
aws ec2 revoke-security-group-ingress --group-id ${{ env.EC2_SECURITY_GROUP_ID }} --protocol tcp --port 22 --cidr ${{ steps.ip.outputs.ipv4 }}/32